Tick bars
24 June 2021
In this article we are going through a tick bars calculation and adjustment with corporate actions data using our API within our web cloud-based JupyterLab environment.
Bars are widely used in technical analysis, here are few use-cases:
- Visualize time series and identify market trends
- Feed strategy and decision making bots
- Enrich investment decision making parameters
Depending on the traded asset, its volatility and how latency-sensitive your strategy is, you might use different bar durations: 1min, 5 min...
Building bespoke tick bars on-the-fly from raw vendor tick data can be time consuming and costly. In this article we are explaining how to request custom tick bars data using Ganymede, our web and cloud-based JupyterLab environment, and our API.
Getting started
A rich set of analytics is available on various languages (Python, C#, F#...). For this example, we assume you will use Python using the following package:
We will be using a set of Systemathics modules in addition to Opensource modules as follows:
# open source modules
import os
import grpc
from datetime import datetime
import google.type.date as date
import google.protobuf.duration as duration
# systemathics modules to handle input parameters' types
import systemathics.apis.type.shared.identifier as identifier
import systemathics.apis.type.shared.constraints as constraints
import systemathics.apis.type.shared.date_interval as dateinterval
# systemathics modules to instantiate the tick bars service
import systemathics.apis.services.tick_analytics.tick_bars as tick_bars
import systemathics.apis.services.tick_analytics.tick_bars_grpc as tick_bars_service
Tick bars service belongs to a wide list of analytics made available within our data solutions. The service is called TickBarsService and enables to get tick bars data before and after adjustments with corporate actions data. Explore the full API documentation to learn more about our extensive list of analytics.
Request parameters
# set instrument identifier
ticker = 'AAPL'
exchange = 'BATS'
# set the bar duration, free entry in seconds
my_sampling = 5 * 60
# set the bar calculation field, enumeration: trade, bid, ask
my_field = tick_bars.BAR_PRICE_TRADE
# set the look back period, we are using Google date format
start = date.Date(year = 2020, month = 8, day = 28)
end = date.Date(year = 2020, month = 9, day = 1)
Create the request
Now that we selected the input parameters we proceeed to the request creation:
# build date interval out of input look back period
date_interval = dateinterval.DateInterval(
start_date = date.Date(year = start.year, month = start.month, day = start.day),
end_date = date.Date(year = end.year, month = end.month, day = end.day)
)
# generate tick bars request
my_request = tick_bars.TickBarsRequest(
identifier = identifier.Identifier(exchange = exchange, ticker = ticker),
constraints = constraints.Constraints(date_intervals = [date_interval]),
sampling = duration.Duration(seconds = my_sampling),
field = my_field,
adjustment = False # do not adjust data (split)
)
Process the request
- we are using gRPC channel to overcome performance issues usually encountered when retrieving high volumes of data
- we embed the token within the request. This token is used to grant authorization to a user or a group of users over the time: history depth, tickers watchlist...
# instantiate the tick bars service
my_service = tick_bars_service.TickBarsServiceStub(channel)
# process the tick bars request (unadjusted)
bars = []
my_metadata = [('authorization', token)]
for bar in service.TickBars(request= my_request, metadata = my_metadata):
bars.append(bar)
Retrieve data
# import required modules
import pandas as pd
import mplfinance as mpf
# create a pandas dataframe with: dates, bars and ticks count used for each bar
d = {'Date': dates, 'Open': opens, 'High': highs, 'Low' : lows,'Close': closes, 'Volume': volumes, 'Count': counts}
df = pd.DataFrame(data=d)
df = df.set_index('Date')
df
Note that we chose a look back period including an Apple split(1:4) on 2020-08-31, below 5-min bars for AAPL-BATS before and after split event application. By setting adjustment parameter to True or False you choose to retrieve tick data before or after corporate actions application.
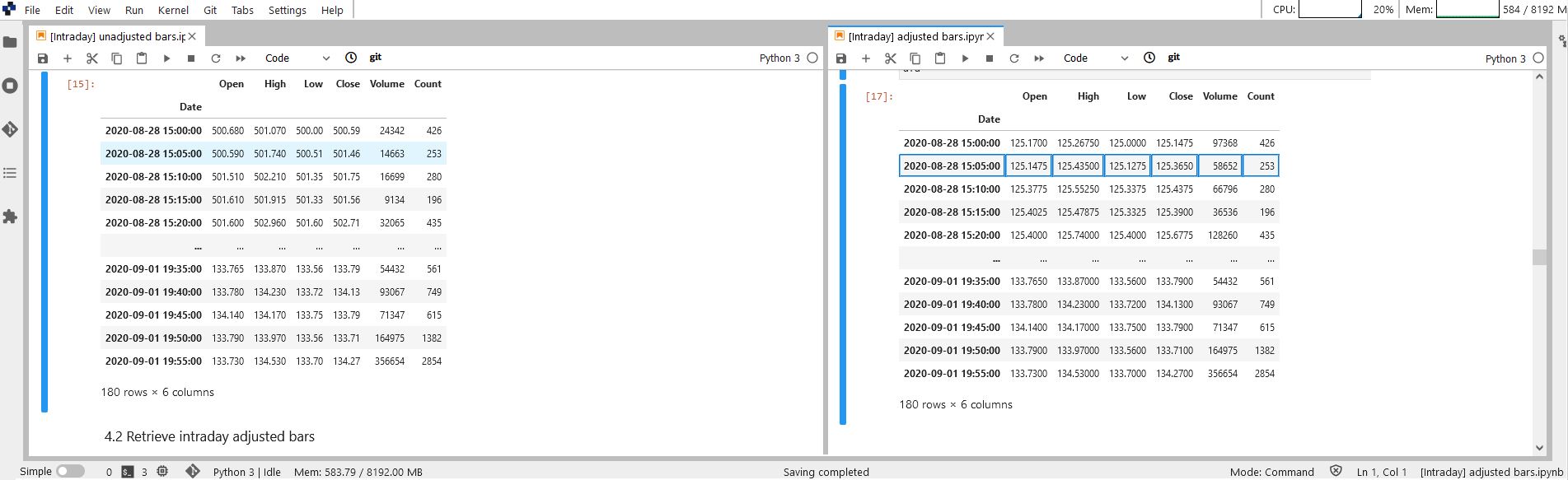
You can use libraries of your choice for graph plotting, in this example we are using an open-source library mplfinance to visualize bar charts.
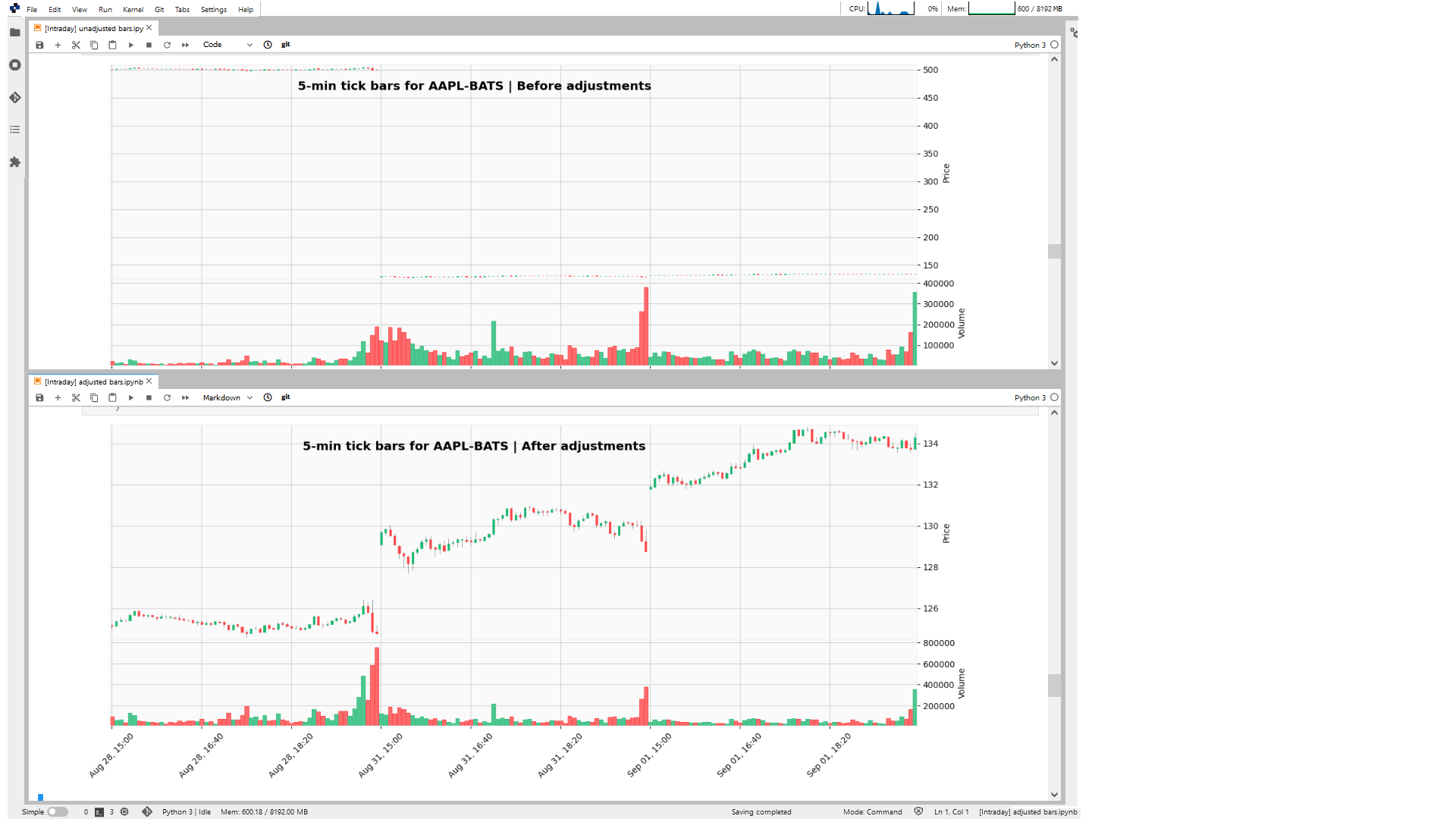
In this article, we request tick bars by calling a dedicated API service within Ganymede, our web and cloud-based JupyterLab environment, and our API. You can use it as-is and/or call directly our API within your internal tools and start immediately retrieving on-demand financial data.
To get the full sample and discover more data analytics samples and building blocks navigate to our public Github →