Multiple assets tick requests
18 January 2022
This article is a quick note on how the user can handle tick data from multiple assets at the same time. We will show the few steps to retrieve tick data from multiple assets in a single request thanks to our web cloud-based JupterLab environment and our API.
In this blog post we will explain the way to handle TickTrades endpoint with multiple instruments at a time. It enables you to request for trades for a given watchlist and any optional time constraints.
The use-cases of retrieving trades and top of the book are multiple:
- Get a snapshot of the market for any best execution scenario
- Generate indicators to highlight market activity
Get started
As we will be using the Systemathics TickTrades endpoint, we need beforehand to import that module alongside with some helper and open source modules.
# open source modules
import grpc
import pandas as pd
from datetime import datetime
# Google modules
import google.type.date_pb2 as date
import google.type.timeofday_pb2 as timeofday
import google.type.dayofweek_pb2 as dayofweek
import google.protobuf.duration_pb2 as duration
# Systemathics helper modules
import systemathics.apis.type.shared.v1.identifier_pb2 as identifier
import systemathics.apis.type.shared.v1.constraints_pb2 as constraints
import systemathics.apis.type.shared.v1.date_interval_pb2 as dateinterval
import systemathics.apis.type.shared.v1.time_interval_pb2 as timeinterval
# Systemathics modules for the TickTrades service
import systemathics.apis.services.tick.v1.tick_trades_pb2 as tick_trades
import systemathics.apis.services.tick.v1.tick_trades_pb2_grpc as tick_trades_service
Request parameters
The first step in order to use the TickTrades endpoint is to define your input parameters. In this example we chose to retrieve trades from an array of two identifiers.
# generate the tick trades request for the different assets
aapl_bats = identifier.Identifier(exchange = "BATS", ticker = "AAPL")
aapl_xngs = identifier.Identifier(exchange = "XNGS", ticker = "AAPL")
If necessary, define some time constraints to filter the span of the snapshot as shown below:
# create time intervals (we are using Google date format)
date_interval = dateinterval.DateInterval(
start_date = date.Date(year = 2021, month = 1, day = 5),
end_date = date.Date(year = 2021, month = 1, day = 5))
# build the market data request time interval
# UTC time zone
time_interval = timeinterval.TimeInterval(
start_time = timeofday.TimeOfDay(hours = 12, minutes = 0, seconds = 3),
end_time = timeofday.TimeOfDay(hours = 12, minutes = 0, seconds = 10))
# generate constraints based on the previous time selection
my_constraints = constraints.Constraints(
date_intervals = [date_interval],
time_intervals = [time_interval],)
Once your inputs defined, you have to create your request with the list of identifiers and your constraints.
# generate the tick trades request with multiple identifiers
request = tick_trades.TickTradesRequest(
identifiers = [aapl_bats, aapl_xngs],
constraints = my_constraints)
Process request
Our API uses a gRPC channel and the response of the TickTrades service is streamed due to the high volumes of data. The code snippet below instantiates the correct service, runs the request and stores the response.
# instantiate the tick trades service
service = tick_trades_service.TickTradesServiceStub(channel)
# process the tick trades request
trades = []
metadata = [('authorization', token)]
for trade in service.TickTrades(request=request, metadata=metadata):
trades.append(trade)
Retrieve data
When requesting the TickTrades endpoint with several instruments, the API streamed response is a series of trade objects:
- The first row is a unique mapping field that the user can use to match the remaining streamed data to the respective instrument. This field is usually ignored when processing one instrument at a time.
- Any following row is a trade containing important financial figures (price, size, timestamp, condition, etc) as well as a tag used to match the current row to the correct asset.
The image below shows the mapping returned in the response for the different instruments:
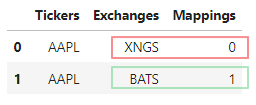
Finally, here are the trades of the response exposed in a dataframe.
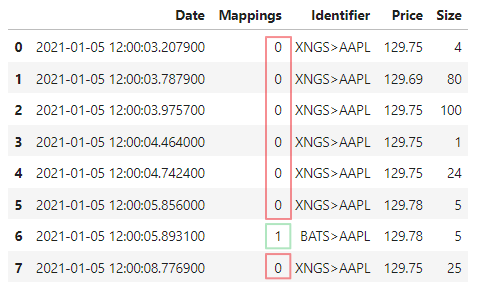
With this feature, you can retrieve a snapshot of the market at a precise moment for a given watchlist, and easily match each trade to the corresponding instrument.
Do not hesitate to contact us to experience this feature through Ganymede, our web and cloud-based JupyterLab environment, and our API.